Thanks a lot for responses and comments to make my small efforts on TinyOS programming a success. So here is another good news for C programmers who wants to work on TinyOS. No more NesC codes and hurdles of it.
TinyOS 2.10 comes with integrated library for tinythreads. The library examples can be found in "/opt/tinyos-2.1.0/apps/tosthreads" for a default installation.
The directory under it, "capps" is specially interesting to us.
Here I assume that you have done TinyOS installation and Avrora configuration from my past posts and enjoyed testing the blink application and blink2radio application.Also you have done programming in Threads using C.
What is so exciting in this folder? Well we are back to our favourite language "C". Let's rewrite the program using C and tosthreads
Quick refresh on Posix standard Thread in C
- Declare thread_t instances
- Define functions of void* foo(void*)
- Create thread using pthread_create()
TOSTHREADS programming
make mica2 cthreadsRevisiting Blink
#include "tosthread.h"
#include "tosthread_leds.h"
tosthread_t blink;
void blink_thread(void* arg);
void tosthread_main(void*arg)
{
tosthread_create(&blink,blink_thread,NULL,400);
}
void blink_thread(void*arg)
{
uint8_t counter;
for(counter=0;counter<8;counter++)
{
setLeds(counter) ; tosthread_sleep(200);
}
}
Save the above code as Blink.c
Making the program : Makefile
TOSTHREAD_MAIN=Blink.c
include $(MAKERULES) Save above code as Makefile
Compiling the program
Open a shell in the same folder where Makefile and Blink.c are stored. Type
#include "tosthread.h"
#include "tosthread_leds.h"
tosthread_t blink;
void blink_thread(void* arg);
void tosthread_main(void*arg)
{
tosthread_create(&blink,blink_thread,NULL,400);
}
void blink_thread(void*arg)
{
uint8_t counter;
for(counter=0;counter<8;counter++)
{
setLeds(counter) ; tosthread_sleep(200);
}
}
Save the above code as Blink.c
Making the program : Makefile
TOSTHREAD_MAIN=Blink.c
include $(MAKERULES) Save above code as Makefile
Compiling the program
Open a shell in the same folder where Makefile and Blink.c are stored. Type
If everything goes fine you will get output similar to
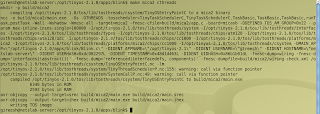
Running the program using Avrora
From shell change directory to "mica2/build"
cd mica2/build
Convert the main.exe to blink.od
convert-avrora main.exe blink.od
Run the simulation
avrora -platform=mica2 -seconds=3 blink.od
Output will be similar to the following
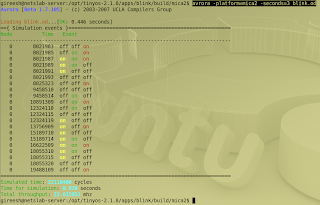
Tip: Modify the program to make the thread running for ever instead of 8 counts.
Keep reading!!!!
I will be back with more programs.
Meanwhile if you have any simple code, please send it or add as comments so that others will be benefited.
Links you might be interested
Running TinyOS Programs using Avrora
Running multiple node simulation using Avrora: Example BlinkToRadio
Installation of TinyOS 2.10 in Ubuntu
WSN: A layman's view